BriteLedgers V1
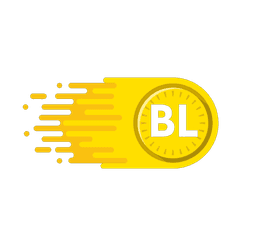
"Managing the Supply Chain With Brilliance"
BriteLedgers stands as the first of its kind, a decentralized supply chain protocol that revolutionizes supply chains. We are aiming to develop a stable, decentralized supply chain management protocol for diverse industries, transforming operations and driving growth
Table of Contents
Core Concept
The BriteLedgers V1 protocol revolutionizes supply chain management through a shared ledger. This transparent and secure solution optimizes demand forecasing, inventory, streamlines operations, and enhances market information. By incentivizing compliance, improving access to finance, and ensuring security, BriteLedgers V1 aims to set new standards in supply chain management.
Proof of Concept
-exac_path: src/contracts
Features
The BriteLedgers protocol includes:
- A comprehensive interface for interacting with the protocol.
- A robust contract codebase written in Solidity.
- Extensive tests to ensure the security and reliability of the protocol.
- Detailed documentation to help you understand and use the protocol.
Getting Started
Setup & Installation
- Clone this repository:
git clone https://github.com/tinotenda01/briteledgers.git
- Set up foundry:
curl -L https://foundry.paradigm.xyz | bash
.
-
This will install Foundryup, then simply follow the instructions on-screen, which will make the
foundryup
command available in your CLI. -
Running
foundryup
by itself will install the latest (nightly) precompiled binaries:forge
,cast
,anvil
, andchisel
. Commandfoundryup --help
for more options, like installing from a specific version or commit. For complete details about foundry See docs
- Set up your default foundry comands inside the makefile
How to Use
Contributing
Contributions are welcomed. If you find a bug or have a feature request, please open an issue. If you would like to contribute code, please open a pull request. For more details, see the CONTRIBUTING.md file.
License
This project is licensed under the GPL License - see the LICENSE.md file for details.
Please note that this project is released with a Contributor Code of Conduct. By participating in this project you agree to abide by its terms.
Contents
Contents
Contents
Commodity
This contract represents a commodity in a supply chain.
It includes functions for managing the commodity's status and the parties involved in its shipment.
State Variables
Owner
address Owner;
description
bytes32 description;
rawMaterials
address[] rawMaterials;
transporters
address[] transporters;
manufacturer
address manufacturer;
wholesaler
address wholesaler;
distributor
address distributor;
finalConsumer
address finalConsumer;
quantity
uint256 quantity;
status
commodityStatus status;
txnContractAddress
address txnContractAddress;
Functions
constructor
Creates a new commodity batch.
constructor(
address _manufacturerAddr,
bytes32 _description,
address[] memory _rawAddr,
uint256 _quantity,
address[] memory _transporterAddr,
address _receiverAddr,
uint256 RcvrType
);
Parameters
Name | Type | Description |
---|---|---|
_manufacturerAddr | address | The address of the manufacturer creating the batch. |
_description | bytes32 | A brief description of the batch. |
_rawAddr | address[] | An array of addresses representing the raw materials used in the batch. |
_quantity | uint256 | The quantity of commodity in the batch. |
_transporterAddr | address[] | An array of addresses representing the transporters involved in the shipment. |
_receiverAddr | address | The address of the receiver of the batch. |
RcvrType | uint256 | An identifier for the type of receiver (1 for wholesaler, 2 for distributor). |
getCommodityInfo
Returns information about the commodity batch.
function getCommodityInfo()
public
view
returns (
address _manufacturerAddr,
bytes32 _description,
address[] memory _rawAddr,
uint256 _quantity,
address[] memory _transporterAddr,
address _distributor,
address _finalConsumer
);
Returns
Name | Type | Description |
---|---|---|
_manufacturerAddr | address | The address of the manufacturer. |
_description | bytes32 | A brief description of the commodity batch. |
_rawAddr | address[] | An array of addresses representing the raw materials used in the batch. |
_quantity | uint256 | The quantity of commodity in the batch. |
_transporterAddr | address[] | An array of addresses representing the transporters involved in the shipment. |
_distributor | address | The address of the distributor. |
_finalConsumer | address | The address of the finalConsumer. |
getWDC
Returns the addresses of the wholesaler, distributor, and finalConsumer.
function getWDC() public view returns (address[3] memory WDP);
Returns
Name | Type | Description |
---|---|---|
WDP | address[3] | An array of 3 addresses representing the wholesaler, distributor, and finalConsumer. |
getBatchIDStatus
Returns the current status of the commodity batch.
function getBatchIDStatus() public view returns (uint256);
Returns
Name | Type | Description |
---|---|---|
<none> | uint256 | A uint representing the current status of the commodity batch. |
pickCommodity
Updates the status of the commodity batch when it is picked up by a transporter.
function pickCommodity(address _transporterAddr) public;
Parameters
Name | Type | Description |
---|---|---|
_transporterAddr | address | The address of the transporter picking up the commodity. |
updateTransporterArray
Adds a transporter to the array of transporters involved in the shipment.
function updateTransporterArray(address _transporterAddr) public;
Parameters
Name | Type | Description |
---|---|---|
_transporterAddr | address | The address of the transporter to add. |
receivedCommodity
Updates the status of the commodity batch when it is received by a wholesaler or distributor.
function receivedCommodity(address _receiverAddr) public returns (uint256);
Parameters
Name | Type | Description |
---|---|---|
_receiverAddr | address | The address of the receiver (either wholesaler or distributor). |
Returns
Name | Type | Description |
---|---|---|
<none> | uint256 | A uint representing the new status of the commodity batch. |
sendWtoD
Updates the distributor address and status of the commodity batch when it is sent from the wholesaler to the distributor.
function sendWtoD(address receiver, address sender) public;
Parameters
Name | Type | Description |
---|---|---|
receiver | address | The address of the distributor. |
sender | address | The address of the wholesaler. |
receivedWtoD
Updates the status of the commodity batch when it is received by the distributor from the wholesaler.
function receivedWtoD(address receiver) public;
Parameters
Name | Type | Description |
---|---|---|
receiver | address | The address of the distributor. |
sendDtoC
Updates the finalConsumer address and status of the commodity batch when it is sent from the distributor to the finalConsumer.
function sendDtoC(address receiver, address sender) public;
Parameters
Name | Type | Description |
---|---|---|
receiver | address | The address of the finalConsumer. |
sender | address | The address of the distributor. |
receivedDtoC
Updates the status of the commodity batch when it is received by the finalConsumer from the distributor.
function receivedDtoC(address receiver) public;
Parameters
Name | Type | Description |
---|---|---|
receiver | address | The address of the finalConsumer. |
Events
ShippmentUpdate
Emitted when there is an update to the shipment status.
event ShippmentUpdate(
address indexed BatchID, address indexed Shipper, address indexed Receiver, uint256 TransporterType, uint256 Status
);
Errors
UnAuthorised_PickUp
error UnAuthorised_PickUp();
Only_Wholesaler_or_Distributor_Authorised
error Only_Wholesaler_or_Distributor_Authorised();
Product_not_picked_up_yet
error Product_not_picked_up_yet();
Wholesaler_is_not_Authorised
error Wholesaler_is_not_Authorised();
Distributor_is_not_Authorised
error Distributor_is_not_Authorised();
FinalConsumer_is_not_Authorised
error FinalConsumer_is_not_Authorised();
Package_at_Manufacturer
error Package_at_Manufacturer();
Enums
commodityStatus
Represents the status of a commodity in the supply chain.
enum commodityStatus {
atManufacturer,
pickedForW,
pickedForD,
deliveredAtW,
deliveredAtD,
pickedForC,
deliveredAtC
}
CommodityD_C
Author: Tinotenda Joe
This contract represents a dispatch and collection model for commodities
State Variables
Owner
Storage for contract owner's address
address Owner;
medAddr
Addresses for medAddr, sender, transporter, receiver
address medAddr;
sender
address sender;
transporter
address transporter;
receiver
address receiver;
status
Store the status of package
packageStatus status;
Functions
constructor
Constructor that initializes contract with initial parameters
constructor(address _address, address Sender, address Transporter, address Receiver);
Parameters
Name | Type | Description |
---|---|---|
_address | address | The medAddr |
Sender | address | The sender's address |
Transporter | address | The transporter's address |
Receiver | address | The receiver's address |
pickDC
Called when the associated transporter initiates this function
function pickDC(address _address, address transporterAddr) public;
Parameters
Name | Type | Description |
---|---|---|
_address | address | The commodity address |
transporterAddr | address | The transporter's address |
receiveDC
Called when the associated receiver initiates this function
function receiveDC(address _address, address Receiver) public;
Parameters
Name | Type | Description |
---|---|---|
_address | address | The commodity address |
Receiver | address | The receiver's address |
get_addressStatus
Returns the current status of the package
function get_addressStatus() public view returns (uint256);
Returns
Name | Type | Description |
---|---|---|
<none> | uint256 | status The current status of the package |
Errors
UnAuthorised_Receiver
error UnAuthorised_Receiver();
Transporter_is_not_Authorised
error Transporter_is_not_Authorised();
Enums
packageStatus
Enumeration of different package statuses
enum packageStatus {
atcreator,
picked,
delivered
}
CommodityW_D
Author: Tinotenda Joe
This contract represents a shipping model for commodities
State Variables
Owner
Store the contract owner's address
address Owner;
commodityId
Addresses for commodityId, sender, transporter, receiver
address commodityId;
sender
address sender;
transporter
address transporter;
receiver
address receiver;
status
Stores the current package status
packageStatus status;
Functions
constructor
Setup the contract with initial details
constructor(address _address, address Sender, address Transporter, address Receiver);
Parameters
Name | Type | Description |
---|---|---|
_address | address | The commodity address |
Sender | address | The sender's address |
Transporter | address | The transporter's address |
Receiver | address | The receiver's address |
pickWD
Called when the transporter picks the package
function pickWD(address _address, address _transporter) public;
Parameters
Name | Type | Description |
---|---|---|
_address | address | The commodity address |
_transporter | address | The transporter's address |
receiveWD
Called when the receiver receives the package
function receiveWD(address _address, address Receiver) public;
Parameters
Name | Type | Description |
---|---|---|
_address | address | The commodity address |
Receiver | address | The receiver's address |
getBatchIDStatus
Returns the current status of the package
function getBatchIDStatus() public view returns (uint256);
Returns
Name | Type | Description |
---|---|---|
<none> | uint256 | status The current status of the package |
Errors
UnAUthorised_Receivers_Account
Thrown when an unauthorized receiver's account is used
error UnAUthorised_Receivers_Account();
UnAUthorised_Shippers_Account
Thrown when an unauthorized shipper's account is used
error UnAUthorised_Shippers_Account();
Enums
packageStatus
Enumeration of various package statuses
enum packageStatus {
atcreator,
picked,
delivered
}
RawMaterial
Author: Tinotenda Joe
This contract is part of a supply chain protocol that allows managing the logistics of raw materials
State Variables
Owner
address Owner;
productid
address productid;
description
bytes32 description;
quantity
uint256 quantity;
transporter
address transporter;
manufacturer
address manufacturer;
supplier
address supplier;
status
packageStatus status;
packageReceiverDescription
bytes32 packageReceiverDescription;
txnContractAddress
address txnContractAddress;
Functions
constructor
Construct a new RawMaterial contract
constructor(
address _creatorAddr,
address _productid,
bytes32 _description,
uint256 _quantity,
address _transporterAddr,
address _manufacturerAddr
);
Parameters
Name | Type | Description |
---|---|---|
_creatorAddr | address | the address of the creator |
_productid | address | the ID of the product |
_description | bytes32 | the description of the product |
_quantity | uint256 | the quantity of the product |
_transporterAddr | address | the address of the transporter |
_manufacturerAddr | address | the address of the manufacturer |
getSuppliedRawMaterials
Returns all the supplied raw materials
function getSuppliedRawMaterials()
public
view
returns (address, bytes32, uint256, address, address, address, address);
Returns
Name | Type | Description |
---|---|---|
<none> | address | productid, description, quantity, supplier, transporter, manufacturer, txnContractAddress |
<none> | bytes32 | |
<none> | uint256 | |
<none> | address | |
<none> | address | |
<none> | address | |
<none> | address |
getRawMaterialStatus
Returns the status of the raw material
function getRawMaterialStatus() public view returns (uint256);
Returns
Name | Type | Description |
---|---|---|
<none> | uint256 | status of the raw material |
pickPackage
Pick a package from the supplier
function pickPackage(address _transporterAddr) public;
Parameters
Name | Type | Description |
---|---|---|
_transporterAddr | address | the address of the transporter |
receivedPackage
Receive a package at the manufacturer
function receivedPackage(address _manufacturerAddr) public;
Parameters
Name | Type | Description |
---|---|---|
_manufacturerAddr | address | the address of the manufacturer |
Events
ShippmentUpdate
Reports a status update for the shipment
event ShippmentUpdate(
address indexed ProductID,
address indexed Transporter,
address indexed Manufacturer,
uint256 TransporterType,
uint256 Status
);
Enums
packageStatus
Possible statuses of a package
enum packageStatus {
atCreator,
picked,
delivered
}
Contents
FinalConsumer
Author: Tinotenda Joe
This contract manages the commodities received by Consumers and track of their sales status
State Variables
CommodityBatchAtFinalConsumer
Tracks the commodities at the Consumer
mapping of Consumer address to array of addresses
mapping(address => address[]) public CommodityBatchAtFinalConsumer;
sale
Tracks the sale status of commodities
mapping of address to sale status
mapping(address => salestatus) public sale;
Functions
commodityRecievedAtFinalConsumer
Function to be called when the Consumer receives a commodity
function commodityRecievedAtFinalConsumer(address _address, address cid) public;
Parameters
Name | Type | Description |
---|---|---|
_address | address | The address of the commodity |
cid | address | The identifier for the commodity |
updateSaleStatus
Function to update the sale status of a commodity
function updateSaleStatus(address _address, uint256 Status) public;
Parameters
Name | Type | Description |
---|---|---|
_address | address | The address of the commodity |
Status | uint256 | The status of the sale |
salesInfo
Function to get sales information for a given commodity
function salesInfo(address _address) public view returns (uint256 Status);
Parameters
Name | Type | Description |
---|---|---|
_address | address | The address of the commodity |
Returns
Name | Type | Description |
---|---|---|
Status | uint256 | The status of the sale |
Events
CommodityStatus
Event to be emitted when commodity status changes
Event includes the commodity address, Consumer's address and status
event CommodityStatus(address _address, address indexed Consumer, uint256 status);
Enums
salestatus
Enumeration for possible statuses of the sale
enum salestatus {
notfound,
atConsumer,
sold,
expired,
damaged
}
Contents
Distributor
Author: Tinotenda Joe
This contract manages commodities received by distributor and their transfer
State Variables
CommoditysAtDistributor
Track commodities at distributor's address
Mapping of distributor's address to array of commodity addresses
mapping(address => address[]) public CommoditysAtDistributor;
CommodityDtoC
Track transfer of commodities from distributor to finalConsumer
Mapping of distributor's address to array of CommodityD_C contract addresses
mapping(address => address[]) public CommodityDtoC;
CommodityDtoCTxContract
Track individual commodity transfer contracts
Mapping of commodity address to CommodityD_C contract address
mapping(address => address) public CommodityDtoCTxContract;
Functions
commodityRecievedAtDistributor
Function to be called when a commodity is received at the distributor
function commodityRecievedAtDistributor(address _address, address cid) public;
Parameters
Name | Type | Description |
---|---|---|
_address | address | The address of the commodity |
cid | address | The identifier for the commodity |
transferCommodityDtoC
Function to transfer commodity from distributor to finalConsumer
function transferCommodityDtoC(address _address, address transporter, address receiver) public;
Parameters
Name | Type | Description |
---|---|---|
_address | address | The address of the commodity |
transporter | address | The transporter's address |
receiver | address | The receiver or finalConsumer's address |
Contents
DLTProvider
Author:
This contract should provide distributed ledger technology (DLT) services to the supply chain stakeholders. It should allow them to read from and write to the ledger in a secure and decentralized manner.
Functions
constructor
constructor();
Ledger
Inherits: Supplier, Transporter, Manufacturer, Wholesaler, Distributor, FinalConsumer
Author: Tinotenda Joe
The contract's core is a 'Tokenized Shared Ledger' that logs every transaction and interaction in the supply chain. This ledger provides an immutable, transparent, and verifiable record of the entire process, from the supplier to the consumer.
The contract can connect with existing off-chain, legacy supply chain systems. It incorporates both on and off-chain security measures to protect sensitive data while maintaining its transparent and traceable nature .
This contract models multiple roles within the supply chain: Supplier, Transporter, Manufacturer, Wholesaler, Distributor, and Consumer. Each role is tied to a unique address and has role-specific functions.
The contract integrates the Industrial Internet of Things (IIOT) for real-time data monitoring and recording at various stages of the supply chain. .
State Variables
Owner
Owner of the contract
address public Owner;
userInfo
Mapping from address to userData
mapping(address => userData) public userInfo;
Functions
constructor
constructor();
onlyOwner
Ensures that only the owner can call the function
modifier onlyOwner();
checkUser
Validates handed-over users
modifier checkUser(address addr);
registerUser
Registers a user with a given name, location, role, and address. Can only be executed by the owner of the contract. Emits a UserRegister event after successful registration.
function registerUser(bytes32 name, string[] memory loc, uint256 role, address _userAddr) external onlyOwner;
Parameters
Name | Type | Description |
---|---|---|
name | bytes32 | user's name |
loc | string[] | user's location |
role | uint256 | user's role |
_userAddr | address | user's address |
changeUserRole
Allows the owner to change the role of a user.
function changeUserRole(uint256 _role, address _addr) external onlyOwner returns (string memory);
Parameters
Name | Type | Description |
---|---|---|
_role | uint256 | The new role of the user. |
_addr | address | The address of the user. |
Returns
Name | Type | Description |
---|---|---|
<none> | string | Status of the operation. |
getUserInfo
Allows the owner to get the user information of the given address.
function getUserInfo(address _address) external view onlyOwner returns (userData memory);
Parameters
Name | Type | Description |
---|---|---|
_address | address | Address of the user. |
Returns
Name | Type | Description |
---|---|---|
<none> | userData | User information. |
supplierCreatesRawPackage
Creates a package of raw material
Can only be executed by a supplier
function supplierCreatesRawPackage(
bytes32 _description,
uint256 _quantity,
address _transporterAddr,
address _manufacturerAddr
) external;
Parameters
Name | Type | Description |
---|---|---|
_description | bytes32 | Description of the raw material |
_quantity | uint256 | Quantity of the raw material |
_transporterAddr | address | Address of the transporter |
_manufacturerAddr | address | Address of the manufacturer |
supplierGetPackageCount
Returns the count of packages created by the supplier
Can only be executed by a supplier
function supplierGetPackageCount() external view returns (uint256);
Returns
Name | Type | Description |
---|---|---|
<none> | uint256 | The number of packages created by the supplier |
supplierGetRawMaterialAddresses
Returns the addresses of all packages created by the supplier
Can only be executed by a supplier
function supplierGetRawMaterialAddresses() external view returns (address[] memory);
Returns
Name | Type | Description |
---|---|---|
<none> | address[] | An array of addresses of all packages created by the supplier |
transporterHandlePackage
Handles the package by the transporter
Can only be executed by a transporter
function transporterHandlePackage(address _address, uint256 transporterType, address cid) external;
Parameters
Name | Type | Description |
---|---|---|
_address | address | The address of the package |
transporterType | uint256 | The type of the transporter |
cid | address | The id of the commodity |
manufacturerReceivedRawMaterials
Handles the receipt of raw materials by the manufacturer
Can only be executed by a manufacturer
function manufacturerReceivedRawMaterials(address _addr) external;
Parameters
Name | Type | Description |
---|---|---|
_addr | address | The address of the raw material package |
manufacturerCreatesNewCommodity
Creates a new commodity by the manufacturer
Can only be executed by a manufacturer
function manufacturerCreatesNewCommodity(
bytes32 _description,
address[] memory _rawAddr,
uint256 _quantity,
address[] memory _transporterAddr,
address _receiverAddr,
uint256 RcvrType
) external returns (string memory);
Parameters
Name | Type | Description |
---|---|---|
_description | bytes32 | The description of the commodity |
_rawAddr | address[] | The addresses of the raw materials |
_quantity | uint256 | The quantity of the commodity |
_transporterAddr | address[] | The addresses of the transporters |
_receiverAddr | address | The address of the receiver |
RcvrType | uint256 | The type of the receiver |
Returns
Name | Type | Description |
---|---|---|
<none> | string | A message indicating the commodity has been created |
wholesalerReceivedCommodity
Handles the receipt of a commodity by the wholesaler
Can only be executed by a wholesaler or a distributor
function wholesalerReceivedCommodity(address _address) external;
Parameters
Name | Type | Description |
---|---|---|
_address | address | The address of the commodity |
transferCommodityW_D
Transfers a commodity from a wholesaler to a distributor
Can only be executed by a wholesaler or the current owner of the package
function transferCommodityW_D(address _address, address transporter, address receiver) external;
Parameters
Name | Type | Description |
---|---|---|
_address | address | The address of the commodity |
transporter | address | The address of the transporter |
receiver | address | The address of the receiver |
getBatchIdByIndexWD
Returns the batch id by index for a wholesaler
Can only be executed by a wholesaler
function getBatchIdByIndexWD(uint256 index) external view returns (address packageID);
Parameters
Name | Type | Description |
---|---|---|
index | uint256 | The index of the batch |
Returns
Name | Type | Description |
---|---|---|
packageID | address | The id of the package |
getSubContractWD
Returns the sub contract for a wholesaler
Can only be executed by a wholesaler
function getSubContractWD(address _address) external view returns (address SubContractWD);
Parameters
Name | Type | Description |
---|---|---|
_address | address | The address of the wholesaler |
Returns
Name | Type | Description |
---|---|---|
SubContractWD | address | The address of the sub contract |
distributorReceivedCommodity
This function is called when a distributor receives a commodity
Only a distributor or the current owner of the package can call this function
function distributorReceivedCommodity(address _address, address cid) external;
Parameters
Name | Type | Description |
---|---|---|
_address | address | The address of the distributor |
cid | address | The commodity id |
distributorTransferCommoditytoFinalConsumer
This function transfers a commodity from a distributor to a final consumer
Only a distributor or the current owner of the package can call this function
function distributorTransferCommoditytoFinalConsumer(address _address, address transporter, address receiver)
external;
Parameters
Name | Type | Description |
---|---|---|
_address | address | The address of the commodity |
transporter | address | The address of the transporter |
receiver | address | The address of the receiver |
getBatchesCountDC
This function returns the count of batches for a distributor
Only a distributor can call this function
function getBatchesCountDC() external view returns (uint256 count);
Returns
Name | Type | Description |
---|---|---|
count | uint256 | The count of batches |
getBatchIdByIndexDC
This function returns the batch id by index for a distributor
Only a distributor can call this function
function getBatchIdByIndexDC(uint256 index) external view returns (address packageID);
Parameters
Name | Type | Description |
---|---|---|
index | uint256 | The index of the batch |
Returns
Name | Type | Description |
---|---|---|
packageID | address | The id of the package |
getSubContractDC
This function returns the sub contract for a distributor
function getSubContractDC(address _address) external view returns (address SubContractDP);
Parameters
Name | Type | Description |
---|---|---|
_address | address | The address of the distributor |
Returns
Name | Type | Description |
---|---|---|
SubContractDP | address | The address of the sub contract |
consumerReceivedCommodity
This function is called when a consumer receives a commodity
Only a consumer can call this function
function consumerReceivedCommodity(address _address, address cid) external;
Parameters
Name | Type | Description |
---|---|---|
_address | address | The address of the consumer |
cid | address | The commodity id |
updateStatus
This function updates the status of a commodity
Only the consumer or the current owner of the package can call this function
function updateStatus(address _address, uint256 Status) external;
Parameters
Name | Type | Description |
---|---|---|
_address | address | The address of the commodity |
Status | uint256 | The new status of the commodity |
getBatchesCountC
This function returns the count of batches for a consumer
Only a wholesaler or the current owner of the package can call this function
function getBatchesCountC() external view returns (uint256 count);
Returns
Name | Type | Description |
---|---|---|
count | uint256 | The count of batches |
getBatchIdByIndexC
This function returns the batch id by index for a consumer
Only a wholesaler or the current owner of the package can call this function
function getBatchIdByIndexC(uint256 index) external view returns (address _address);
Parameters
Name | Type | Description |
---|---|---|
index | uint256 | The index of the batch |
Returns
Name | Type | Description |
---|---|---|
_address | address | The address of the batch |
verify
This function verifies a signature
function verify(address p, bytes32 hash, uint8 v, bytes32 r, bytes32 s) external pure returns (bool);
Parameters
Name | Type | Description |
---|---|---|
p | address | The address that is claimed to be the signer |
hash | bytes32 | The hash of the signed message |
v | uint8 | The recovery id of the signature |
r | bytes32 | The r value of the signature |
s | bytes32 | The s value of the signature |
Returns
Name | Type | Description |
---|---|---|
<none> | bool | bool Whether the signature is valid or not |
Events
UserRegister
Events to make the smart contract interaction transparent
event UserRegister(address indexed _address, bytes32 name);
buyEvent
event buyEvent(address buyer, address seller, address packageAddr, bytes32 signature, uint256 indexed now);
respondEvent
event respondEvent(address buyer, address seller, address packageAddr, bytes32 signature, uint256 indexed now);
sendEvent
event sendEvent(address seller, address buyer, address packageAddr, bytes32 signature, uint256 indexed now);
receivedEvent
event receivedEvent(address buyer, address seller, address packageAddr, bytes32 signature, uint256 indexed now);
Structs
userData
Struct to define a user
struct userData {
bytes32 name;
string[] userLoc;
roles role;
address userAddr;
}
Enums
roles
Enum which defines the different actors in the system
enum roles {
noRole,
supplier,
transporter,
manufacturer,
wholesaler,
distributor,
consumer
}
Contents
Manufacturer
Author: Anonymous
This contract manages the receipt and creation of commodities by a manufacturer
State Variables
manufacturerRawMaterials
Track the raw materials owned by manufacturers
Mapping of manufacturer's address to array of RawMaterial contract addresses
mapping(address => address[]) public manufacturerRawMaterials;
manufacturerCommodities
Track the commodities produced by manufacturers
Mapping of manufacturer's address to array of Commodity contract addresses
mapping(address => address[]) public manufacturerCommodities;
Functions
manufacturerReceivedPackage
Function called when a manufacturer receives a package of raw materials
function manufacturerReceivedPackage(address _addr, address _manufacturerAddress) public;
Parameters
Name | Type | Description |
---|---|---|
_addr | address | The address of the RawMaterial contract |
_manufacturerAddress | address | The address of the manufacturer |
manufacturerCreatesCommodity
Function called when a manufacturer creates a new commodity
function manufacturerCreatesCommodity(
address _manufacturerAddr,
bytes32 _description,
address[] memory _rawAddr,
uint256 _quantity,
address[] memory _transporterAddr,
address _recieverAddr,
uint256 RcvrType
) public;
Parameters
Name | Type | Description |
---|---|---|
_manufacturerAddr | address | The address of the manufacturer |
_description | bytes32 | The description of the commodity |
_rawAddr | address[] | The array of addresses of raw materials used for the commodity |
_quantity | uint256 | The quantity of the commodity |
_transporterAddr | address[] | The array of addresses of transporters for the commodity |
_recieverAddr | address | The address of the receiver of the commodity |
RcvrType | uint256 | The type of the receiver (encoded as a uint256) |
Supplier
Author: Tinotenda Joe
This contract serves as a decentralized platform for suppliers to manage their raw materials in a supply chain protocol.
Following is just a simple explanation, consider implementing more complex logic in accordance with your needs.
State Variables
supplierRawMaterials
mapping(address => address[]) public supplierRawMaterials;
Functions
createRawMaterialPackage
Create a new raw material package
Constructor to set the initial storage
Ensure the proper access controls and error handling (if needed), add events to capture the important state changes TODO: Require checks can be added for inputs
function createRawMaterialPackage(
bytes32 _description,
uint256 _quantity,
address _transporterAddr,
address _manufacturerAddr
) public;
Parameters
Name | Type | Description |
---|---|---|
_description | bytes32 | the description of the raw material |
_quantity | uint256 | the quantity of the raw material |
_transporterAddr | address | the address of the transporter |
_manufacturerAddr | address | the address of the manufacturer |
getNoOfPackagesOfSupplier
Get the number of packages of a supplier
This can be used to quickly check how many packages a supplier has TODO: Consider returning more detailed information about each package
function getNoOfPackagesOfSupplier() public view returns (uint256);
Returns
Name | Type | Description |
---|---|---|
<none> | uint256 | the number of packages of the supplier |
getAllPackages
Get all packages of a supplier
Access controls can be added here to restrict this sensitive information TODO: Access Controls using OpenZeppelin's AccessControl.sol TODO: Consider returning more detailed information about each package
function getAllPackages() public view returns (address[] memory);
Returns
Name | Type | Description |
---|---|---|
<none> | address[] | an array of addresses of the packages of the supplier |
Events
RawMaterialCreated
event RawMaterialCreated(address indexed rawMaterialAddress, address indexed supplier);
Wholesaler
Author: Your Name
This contract manages the transfer of commodities from a wholesaler to a distributor.
This contract interacts with the Commodity and CommodityW_D contracts.
State Variables
CommoditiesAtWholesaler
Maps a wholesaler's address to an array of commodity addresses that the wholesaler has received.
mapping(address => address[]) public CommoditiesAtWholesaler;
CommodityWtoD
Maps a wholesaler's address to an array of CommodityW_D contract addresses that the wholesaler has initiated.
mapping(address => address[]) public CommodityWtoD;
CommodityWtoDTxContract
Maps a commodity's address to the CommodityW_D contract address that manages its transfer from a wholesaler to a distributor.
mapping(address => address) public CommodityWtoDTxContract;
Functions
commodityRecievedAtWholesaler
Marks a commodity as received by the wholesaler.
Calls the receivedCommodity function of the Commodity contract.
function commodityRecievedAtWholesaler(address _address) public;
Parameters
Name | Type | Description |
---|---|---|
_address | address | The address of the Commodity contract. |
transferCommodityWtoD
Initiates the transfer of a commodity from the wholesaler to a distributor.
Creates a new CommodityW_D contract for the transfer.
function transferCommodityWtoD(address _address, address transporter, address receiver) public;
Parameters
Name | Type | Description |
---|---|---|
_address | address | The address of the Commodity contract. |
transporter | address | The address of the transporter. |
receiver | address | The address of the distributor. |
getCommoditiesAtWholesaler
function getCommoditiesAtWholesaler(address _wholesaler) external view returns (address[] memory);
getCommodityWtoD
function getCommodityWtoD(address _wholesaler) external view returns (address[] memory);
Contents
Transactions
Author: Tinotenda Joe
This contract serves as a ledger for recording transactions within a supply chain. /// Logic here keeps track of custody : Owner1 -> Owner2 -> Owner3 -> Owner4 -> Owner5 -> ...
State Variables
Owner
This holds as the first initiator of a specific transaction / exchange in the supply chain. eg creator or supplier of the raw materials, later own can be traced back.But this is not limited to only a single owner, decentralized nature of this transaction ledger will enable any participant to hold the position as an 'Owner' that is any current entity in custody of the comodities
address public Owner;
transactionCount
uint256 public transactionCount;
txns
mapping(uint256 => transactions) public txns;
Functions
constructor
constructor(address _ownerAddress);
createTransactionEntry
Increases the total transaction count and emits an event at the end. Validates previous transaction for all transactions other than the first one.
Establishes a new transaction entry in the ledger
function createTransactionEntry(
bytes32 _transactionHash,
address _from,
address _to,
bytes32 _prevTransaction,
string memory _latitude,
string memory _longitude
) public;
Parameters
Name | Type | Description |
---|---|---|
_transactionHash | bytes32 | Id of the transaction |
_from | address | Source address of the transaction |
_to | address | Destination address for the transaction |
_prevTransaction | bytes32 | Hash of the previous transaction to maintain continuity |
_latitude | string | Latitude of the transaction |
_longitude | string | Longitude of the transaction |
getAllTransactions
Fetch all the transaction entries recorded in the ledger
function getAllTransactions() public view returns (transactions[] memory);
Returns
Name | Type | Description |
---|---|---|
<none> | transactions[] | Array containing all the transactions in the ledger |
getAllTransactionsCount
Retrieves the total count of transactions in the ledger
function getAllTransactionsCount() public view returns (uint256);
Returns
Name | Type | Description |
---|---|---|
<none> | uint256 | Total count of transactions |
getTransaction
Fetches the details of a specific transaction by its index
function getTransaction(uint256 id)
public
view
returns (bytes32, address, address, bytes32, string memory, string memory, uint256);
Parameters
Name | Type | Description |
---|---|---|
id | uint256 | Index of the transaction to fetch |
Returns
Name | Type | Description |
---|---|---|
<none> | bytes32 | Transaction details: hash, source address, destination address, previous transaction, latitude, longitude, timestamp |
<none> | address | |
<none> | address | |
<none> | bytes32 | |
<none> | string | |
<none> | string | |
<none> | uint256 |
Events
transactionCreated
Event emitted once a transaction gets created successfully
event transactionCreated(
bytes32 _transactionHash, address _from, address _to, bytes32 _prevTransaction, string _latitude, string _longitude
);
Errors
TRANSACTION_ERROR_OCCURRED
tracks erros. thrown from createTransactionEntry
fx when there is a transaction related error during entry creation
error TRANSACTION_ERROR_OCCURRED();
Structs
transactions
Represents a transaction in the ledger with respective details
struct transactions {
bytes32 transactionHash;
address fromAddr;
address toAddr;
bytes32 prevTransaction;
string latitude;
string longitude;
uint256 timestamp;
}
Contents
Transporter
Author: Tinotenda Joe
This contract manages the handling of packages in the supply chain
Functions
handlePackage
Function to handle picking of packages
function handlePackage(address _addr, uint256 transportertype, address cid) public;
Parameters
Name | Type | Description |
---|---|---|
_addr | address | The address of the package origin |
transportertype | uint256 | The type of transporter (1- Supplier to Manufacturer, 2 - Manufacturer to Wholesaler, 3 - Wholesaler to Distributer, 4 - Distributer to finalConsumer) |
cid | address | The identifier for the commodity |
Contents
Wallet
Author:
This contract should manage the funds of each stakeholder in the supply chain. It should allow stakeholders to deposit, withdraw, and transfer funds. It should also handle any tokenomics of the protocol.
Functions
constructor
constructor();
Contents
DemandForecasting
Author: Tinotenda Joe
This contract is used for forecasting demand using historical data. It uses the ABDKMath64x64 library for fixed point arithmetic operations. The contract allows stakeholders to add demand data, calculate forecasted demand, and retrieve demand data.
This contract heavily relies on the ABDKMath64x64 library for fixed point arithmetic operations. The contract uses a moving average approach with optimized alpha for forecasting. The alpha value is optimized using the gradient descent algorithm. The contract also uses linear regression to determine the underlying trend in the historical data.
State Variables
historicalDemand
mapping(uint256 => DemandEntry) historicalDemand;
demandCount
uint256 demandCount;
forecastedDemand
int128 forecastedDemand;
MAX_ITERATIONS
uint256 public MAX_ITERATIONS = 1000;
MIN_ALPHA
int128 public MIN_ALPHA;
MAX_ALPHA
int128 public MAX_ALPHA;
LEARNING_RATE
int128 public LEARNING_RATE;
INITIAL_ALPHA
int128 public INITIAL_ALPHA;
Functions
constructor
Constructor for the DemandForecasting contract
Initializes the MIN_ALPHA, MAX_ALPHA, LEARNING_RATE, and INITIAL_ALPHA values
constructor();
addDemandData
Add new demand data
This function allows stakeholders to add new demand data to the library.
function addDemandData(uint256 _demand) external;
Parameters
Name | Type | Description |
---|---|---|
_demand | uint256 | The demand value to be added. |
calculateForecastedDemand
Calculates the forecasted demand using the moving average approach with optimized alpha.
This function calculates the forecasted demand based on historical demand data using a moving average approach. It first optimizes the alpha value by calling the internal function _optimizeAlpha, passing the demandCount. The optimized alpha is then used to calculate the moving average by calling the internal function _calculateMovingAverage.
function calculateForecastedDemand() external;
_calculateMovingAverage
Calculates the moving average forecast using Exponential Smoothing and linear regression.
This function calculates the moving average forecast for a specified number of periods (_n) based on historical demand data.
The historical demand data must be added using the addDemandData function before calling this function.
function _calculateMovingAverage(uint256 _n, int128 _alpha) internal;
Parameters
Name | Type | Description |
---|---|---|
_n | uint256 | The number of periods to consider for the moving average calculation. |
_alpha | int128 | The smoothing factor used in the Exponential Smoothing calculation. The function initializes the forecast with the latest demand value from the historical data. It then performs linear regression on the historical demand data to determine the underlying trend. Afterward, it applies Exponential Smoothing to adjust the forecast based on the trend and the smoothing factor. |
_optimizeAlpha
Optimizes the alpha value for forecasting using the gradient descent algorithm.
This function optimizes the alpha value for forecasting using the gradient descent algorithm.
function _optimizeAlpha(uint256 _n) internal view returns (int128 optimizedAlpha);
Parameters
Name | Type | Description |
---|---|---|
_n | uint256 | The number of periods to consider for optimization. |
Returns
Name | Type | Description |
---|---|---|
optimizedAlpha | int128 | The optimized alpha value. |
max
function max(int128 a, int128 b) internal pure returns (int128);
min
function min(int128 a, int128 b) internal pure returns (int128);
_optimizeForecast
Applies exponential smoothing by combining the weighted average of the historical demand and the adjusted forecasted demand.
This function applies exponential smoothing by combining the weighted average of the historical demand and the adjusted forecasted demand.
function _optimizeForecast(int128 alpha, uint256 _n) internal view returns (int128 forecastError);
Parameters
Name | Type | Description |
---|---|---|
alpha | int128 | The smoothing factor used to adjust the weights of historical and forecasted demand. |
_n | uint256 | The number of historical periods to consider for the forecast. |
Returns
Name | Type | Description |
---|---|---|
forecastError | int128 | The forecast error calculated based on the optimized alpha value. |
_calculateForecastError
Calculates the forecast error based on the forecasted demand and the actual demand.
This function calculates the forecast error based on the forecasted demand and the actual demand.
function _calculateForecastError(int128 _interimForecastedDemand, int128 _actualDemand)
internal
pure
returns (int128 _interimForecastError);
Parameters
Name | Type | Description |
---|---|---|
_interimForecastedDemand | int128 | The forecasted demand value. |
_actualDemand | int128 | The actual demand value. |
Returns
Name | Type | Description |
---|---|---|
_interimForecastError | int128 | The forecast error calculated as a percentage. |
absValue
function absValue(int128 x) public pure returns (int128);
_calculateLinearRegression
Calculates the linear regression parameters (slope and intercept) based on the specified number of periods.
*The function uses the formula for simple linear regression:
- The formula for the slope (m) and intercept (b) in a linear regression model is: m = (NΣXY - ΣXΣY) / (NΣX^2 - (ΣX)^2) b = (ΣY - mΣX) / N
- Where:
- N is the number of historical periods considered for the linear regression
- ΣXY is the sum of the product of X and Y
- ΣX is the sum of X the x-values (indices) of the historical periods.
- ΣY is the sum of Y the y-values (demand) of the historical periods.
- ΣX^2 is the sum of the squares of X
- The slope represents the change in demand per unit change in the index, and the intercept represents the demand when the index is zero.*
function _calculateLinearRegression(uint256 _n) public view returns (int128 slope, int128 intercept);
Parameters
Name | Type | Description |
---|---|---|
_n | uint256 | The number of historical periods to consider for the linear regression. |
Returns
Name | Type | Description |
---|---|---|
slope | int128 | The slope of the linear regression line. slope = (n * sumXY - sumX * sumY) / (n * sumX2 - sumX * sumX) |
intercept | int128 | The intercept of the linear regression line.intercept = (sumY - slope * sumX) / n |
getDemandCount
Returns the total number of demand data entries.
This function returns the total number of demand data entries.
function getDemandCount() external view returns (uint256);
Returns
Name | Type | Description |
---|---|---|
<none> | uint256 | The total number of demand data entries. |
getHistoricalDemand
Returns the demand and cumulative sum for a specific index.
This function returns the demand and cumulative sum for a specific index.
function getHistoricalDemand(uint256 index) external view returns (string memory, string memory);
Parameters
Name | Type | Description |
---|---|---|
index | uint256 | The index of the demand data entry. |
Returns
Name | Type | Description |
---|---|---|
<none> | string | The demand and cumulative sum for the specified index. |
<none> | string |
uintToString
function uintToString(uint256 v) internal pure returns (string memory);
getLatestDemand
Returns the latest demand data entry.
This function returns the latest demand data entry.
function getLatestDemand() external view returns (uint256);
Returns
Name | Type | Description |
---|---|---|
<none> | uint256 | The latest demand data entry. |
getForecastedDemand
Returns the forecasted demand.
This function returns the forecasted demand.
function getForecastedDemand() external view returns (uint256);
Returns
Name | Type | Description |
---|---|---|
<none> | uint256 | The forecasted demand. |
getMaxIterations
Returns the maximum number of iterations for the alpha optimization.
This function returns the maximum number of iterations for the alpha optimization.
function getMaxIterations() external view returns (uint256);
Returns
Name | Type | Description |
---|---|---|
<none> | uint256 | The maximum number of iterations for the alpha optimization. |
getMinAlpha
Returns the minimum alpha value.
This function returns the minimum alpha value.
function getMinAlpha() external view returns (uint256);
Returns
Name | Type | Description |
---|---|---|
<none> | uint256 | The minimum alpha value. |
getMaxAlpha
Returns the maximum alpha value.
This function returns the maximum alpha value.
function getMaxAlpha() external view returns (uint256);
Returns
Name | Type | Description |
---|---|---|
<none> | uint256 | The maximum alpha value. |
getLearningRate
Returns the learning rate.
This function returns the learning rate.
function getLearningRate() external view returns (uint256);
Returns
Name | Type | Description |
---|---|---|
<none> | uint256 | The learning rate. |
getInitialAlpha
Returns the initial alpha value.
This function returns the initial alpha value.
function getInitialAlpha() external view returns (uint256);
Returns
Name | Type | Description |
---|---|---|
<none> | uint256 | The initial alpha value. |
testCalculateMovingAverage
function testCalculateMovingAverage(uint256 _n, int128 _alpha) public;
testOptimizeAlpha
function testOptimizeAlpha(uint256 _n) public view returns (int128);
testOptimizeForecast
function testOptimizeForecast(int128 alpha, uint256 _n) public view returns (int128);
testCalculateForecastError
function testCalculateForecastError(int128 _interimForecastedDemand, int128 _actualDemand)
public
pure
returns (int128);
Errors
INVALID_ENTRY_INDEX
error INVALID_ENTRY_INDEX();
INVALID_NUMBER_OF_PERIODS
error INVALID_NUMBER_OF_PERIODS();
INVALID_ACTUAL_DEMAND
error INVALID_ACTUAL_DEMAND();
NO_DEMAND_DATA
error NO_DEMAND_DATA();
DEMAND_MUST_BE_GREATER_THAN_ZERO
error DEMAND_MUST_BE_GREATER_THAN_ZERO();
Structs
DemandEntry
struct DemandEntry {
int128 demand;
int128 cumulativeSum;
}
InventoryOptimization
State Variables
IM
mapping(uint256 => uint256) public IM;
ID
mapping(uint256 => uint256) public ID;
IR
mapping(uint256 => uint256) public IR;
SM
mapping(uint256 => uint256) public SM;
SD
mapping(uint256 => uint256) public SD;
SR
mapping(uint256 => uint256) public SR;
P
mapping(uint256 => uint256) public P;
C
mapping(uint256 => uint256) public C;
demandForecasting
DemandForecasting public demandForecasting;
Functions
constructor
constructor(address _demandForecasting);
getForecastedDemand
function getForecastedDemand() public view returns (uint256);